In Visual Studio 2012 onwards , there is a new project template called Unit Test Library (Windows Store apps). A Unit Test Library can contain many test classes.Test classes are marked with the TestClass attribute. Test classes contain test methods, initialization code, and cleanup code.
Test method — Test methods are marked with the TestMethod attribute. These methods run
separately one by one. Each method has a result that determines whether the test was successful,
or if it failed.
Initialization — Every test class can have a single method decorated with the TestInitialize
attribute. This method runs before every test method to perform any intitialization needed. Unit
tests should not use real data, network connections, or database access. If a component needs
a data source, it is best to create a fake one. You can do this in the initialization stage so that
every test method can use it.
Cleanup — Every test class can have a single method decorated with the TestCleanup attribute.
This method runs after every test method to clean up any changes after the test methods.
You can ensure that every test method runs on the same environment and configuration.
This unit test is running on user defined data set and it running with application build state. Then no need to run the application to test it. just Build is enough :)
Lets start.,
** Actually we are gonna test this add() code unit.
3. Then R-click on the Solution and Add-> new Project -> select Unit Test Library and add name to it.
4. Then go to the Unit test project and R-Click on the References from Reference Manager select Solution -> Project and select your project
5. Then create property for unitTest class , It should be type of object that you gonna test
6. Then initialize the unit test .
7. Then Create Test Method
8. Then build the solution
If you can't see the Test Explorer then go to Test -> Windows -> Test Explorer
Here is the results
1. I change add method in Cal class in to this
then our test should be false ...
result with default Cal class..
Enjoy :)
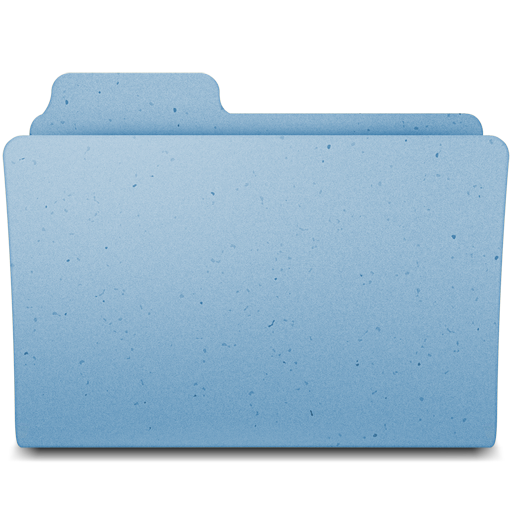
Test method — Test methods are marked with the TestMethod attribute. These methods run
separately one by one. Each method has a result that determines whether the test was successful,
or if it failed.
Initialization — Every test class can have a single method decorated with the TestInitialize
attribute. This method runs before every test method to perform any intitialization needed. Unit
tests should not use real data, network connections, or database access. If a component needs
a data source, it is best to create a fake one. You can do this in the initialization stage so that
every test method can use it.
Cleanup — Every test class can have a single method decorated with the TestCleanup attribute.
This method runs after every test method to clean up any changes after the test methods.
You can ensure that every test method runs on the same environment and configuration.
This unit test is running on user defined data set and it running with application build state. Then no need to run the application to test it. just Build is enough :)
Lets start.,
** Actually we are gonna test this add() code unit.
3. Then R-click on the Solution and Add-> new Project -> select Unit Test Library and add name to it.
4. Then go to the Unit test project and R-Click on the References from Reference Manager select Solution -> Project and select your project
5. Then create property for unitTest class , It should be type of object that you gonna test
6. Then initialize the unit test .
7. Then Create Test Method
8. Then build the solution
If you can't see the Test Explorer then go to Test -> Windows -> Test Explorer
Here is the results
1. I change add method in Cal class in to this
then our test should be false ...
result with default Cal class..
Enjoy :)
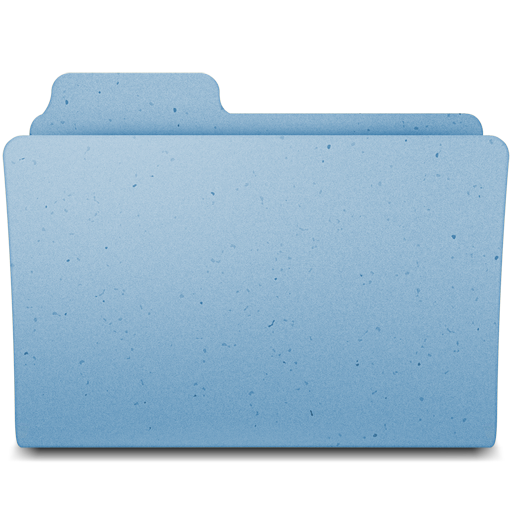
Full Code In Media Fire
http://bit.ly/1blHlHA
0 comments:
Post a Comment