Friday, March 14, 2014
Working with LiveSDK
Live SDK enables you to make connect users in to Live account.
As a developer you can access each logged users
In this post i'm gonna explain how to use Live SDK and demo about how to get Contacts :) As a developer you can access each logged users
- Contacts.
- Calender.
- Sky drive (one drive).
Here we go,
First You need to have Live App
To create live app goto http://msdn.microsoft.com/en-US/live/ and in there in My Apps tab create your own app .. We need CLIENT_ID for our Mobile Application.
Installing LiveSDK
Create New Windows Phone Databound App
Go to Tools -> Nuget Package Manager -> Package Manager Console In the console then type
Install – Package LiveSDK
Or
You can use Visual Studio Extension Manager and search LiveSDK and install
Add Login Button to Application
Go to XAML page that yo want to add Login Button and Paste this inside the Header.
xmlns:live="clr-namespace:Microsoft.Live.Controls;assembly=Microsoft.Live.Controls"
Then add your XAML Button from this code .(Replace CLIENT_ID to your App's CLIENT_ID)
<live:SignInButton Scopes="wl.basic wl.signin " ClientId="CLIENT_ID" Branding="MicrosoftAccount" SessionChanged="SignInButton_SessionChanged" VerticalContentAlignment="Top" Height="100" Margin="-10,0,10,497" VerticalAlignment="Bottom"/>
Scopes ??
Scopes define what kind of data that you can access through your app. This link provide detailed summery of Scopes in Live Client
http://msdn.microsoft.com/en-us/library/live/hh243646.aspx
Scopes define what kind of data that you can access through your app. This link provide detailed summery of Scopes in Live Client
http://msdn.microsoft.com/en-us/library/live/hh243646.aspx
**In My case for just access the contacts I don't need any extended scopes just wl.basic wl.signin is enough.
assume you need to access user calender for that you need to add another field to Scopes as wl.calendars
Then Add This Code part to your C#
private async void SignInButton_SessionChanged(object sender, Microsoft.Live.ControlsLiveConnectSessionChangedEventArgs e)
{
if (e.Status == LiveConnectSessionStatus.Connected)
{
client = new LiveConnectClient(e.Session);
try
{
LiveOperationResult result = await client.GetAsync("me/contacts");
dynamic opresult = result.Result;
List<contact> contactList = new List<contact >();
foreach (var item in opresult.data)
{
contact dummy = new contact();
dummy.Name = item.name;
contactList.Add(dummy);
}
MainLongListSelector.ItemsSource = contactList;
}
catch
{
}
}
}
then Replace your MainPage phone:LongListSelector's Code with this.
<phone:LongListSelector x:Name="MainLongListSelector" Margin="0,150,0,0" ItemsSource="{Binding }" >
<phone:LongListSelector.ItemTemplate>
<DataTemplate>
<StackPanel Margin="0,0,0,17">
<TextBlock Text="{Binding Name}" TextWrapping="Wrap" Style="{StaticResource PhoneTextExtraLargeStyle}"/>
</StackPanel>
</DataTemplate>
</phone:LongListSelector.ItemTemplate>
</phone:LongListSelector>
We are done.. compile and check :)
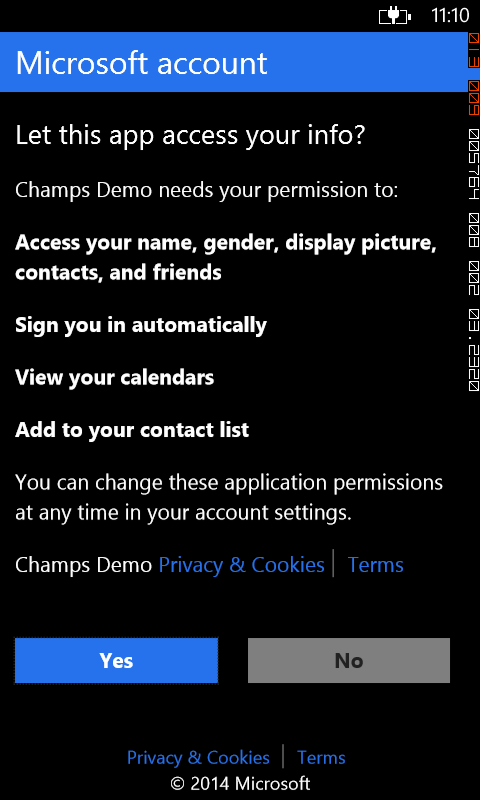
Thanks ...
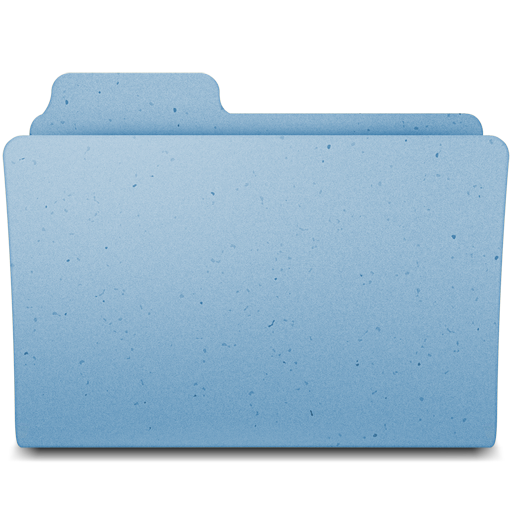
Full Code In Media Fire
http://goo.gl/UrRjY7
Monday, March 10, 2014
Making HTTP Post Request
Here is the correct way to send POST using HTTP Client
var client = new HttpClient();
var postData = new List<KeyValuePair<string, string>>();
postData.Add(new KeyValuePair<string, string>("param1", "value"));
postData.Add(new KeyValuePair<string, string>("param2", "value"));
HttpContent content = new FormUrlEncodedContent(postData);
var response = await client.PostAsync("Post URL", content);
Sunday, March 2, 2014
Working with JSON and HTTP requests
In this post I'm gonna demonstrate how to working with HTTP requests and JSON in Windows Phone and Windows store Applications.
I'm demonstrating Windows Phone App here, there is no different withe method if you are in the Windows Store app either.
1. Create new Windows phone App. I'm using default Windows Phone Data bound App template here.
2. Then Go to the ViewModels -> MainViewModel in the Solution Explorer and find the
and clear all codes in it.
3. We are going to use iTune search Service.. Here is the Http GET url for it. You can try it with your browser too
http://itunes.apple.com/search?term=metallica
4. To handle Http Requests we need to install additional Nuget Package in to the project.
Tools -> Nuget Package Manager -> Package Manager Console
In the console then type
Then Press enter.. You need internet connection to install it. I'll post later abut Nugets
5. Then change LoadData() as async
for get the response from the Service use following code
6. Response cumming as a string we need to convert it to Objects , it will helpful to manage it
for that install Newtonsoft.Json Package in Nuget Package Manager as same as Microsoft.Net.Http
then de-serialize the string in to code... we need classes to convert this string to objects.
7. For that, get the Json response string . (Put break point below this line)
Copy it and
go to the http://json2csharp.com/
paste and click on Generate It will give You class set that you need to de-serialize Json
Add those classes to the namespace I changed RootObject to songRoot
8. Here is the code part for de-serializing
9. We are done.. Then bind the data as you want.. Here is my total code in LoadData(). None of other codes are change.
Enjoy
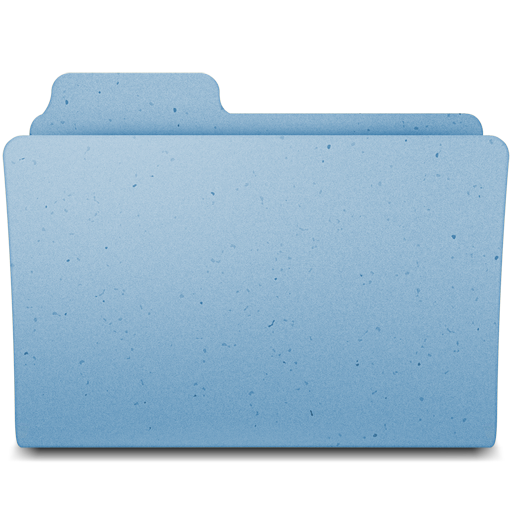
I'm demonstrating Windows Phone App here, there is no different withe method if you are in the Windows Store app either.
1. Create new Windows phone App. I'm using default Windows Phone Data bound App template here.
2. Then Go to the ViewModels -> MainViewModel in the Solution Explorer and find the
public void LoadData()
3. We are going to use iTune search Service.. Here is the Http GET url for it. You can try it with your browser too
http://itunes.apple.com/search?term=metallica
4. To handle Http Requests we need to install additional Nuget Package in to the project.
Tools -> Nuget Package Manager -> Package Manager Console
In the console then type
Install-Package Microsoft.Net.Http
Then Press enter.. You need internet connection to install it. I'll post later abut Nugets
5. Then change LoadData() as async
public async void LoadData()
for get the response from the Service use following code
public async void LoadData()
{
// Sample data; replace with real data
//this.Items.Add(new ItemViewModel() { ID = "0", LineOne = "runtime one", LineTwo = "Maecenas praesent accumsan bibendum", LineThree = "Facilisi faucibus habitant inceptos interdum lobortis nascetur pharetra placerat pulvinar sagittis senectus sociosqu" });
HttpClient client = new HttpClient();
string jsonResponse = await client.GetStringAsync("http://itunes.apple.com/search?term=metallica");
6. Response cumming as a string we need to convert it to Objects , it will helpful to manage it
for that install Newtonsoft.Json Package in Nuget Package Manager as same as Microsoft.Net.Http
PM> Install-Package Newtonsoft.Json
then de-serialize the string in to code... we need classes to convert this string to objects.
7. For that, get the Json response string . (Put break point below this line)
string jsonResponse = await client.GetStringAsync("http://itunes.apple.com/search?term=metallica");
Copy it and
go to the http://json2csharp.com/
paste and click on Generate It will give You class set that you need to de-serialize Json
Add those classes to the namespace I changed RootObject to songRoot
8. Here is the code part for de-serializing
songRoot deserialized = await JsonConvert.DeserializeObjectAsync<songRoot>(jsonResponse);
9. We are done.. Then bind the data as you want.. Here is my total code in LoadData(). None of other codes are change.
public async void LoadData()
{
HttpClient client = new HttpClient();
string jsonResponse = await client.GetStringAsync("http://itunes.apple.com/search?term=metallica");
//for deserialize json and get the class
songRoot deserialized = await JsonConvert.DeserializeObjectAsync<songRoot>(jsonResponse);
foreach (var item in deserialized.results)
{
this.Items.Add(new ItemViewModel()
{
ID = item.trackId.ToString(),
LineOne = item.trackName,
LineTwo = item.trackPrice.ToString(),
LineThree = item.trackCount.ToString(),
});
}
this.IsDataLoaded = true;
}
Enjoy
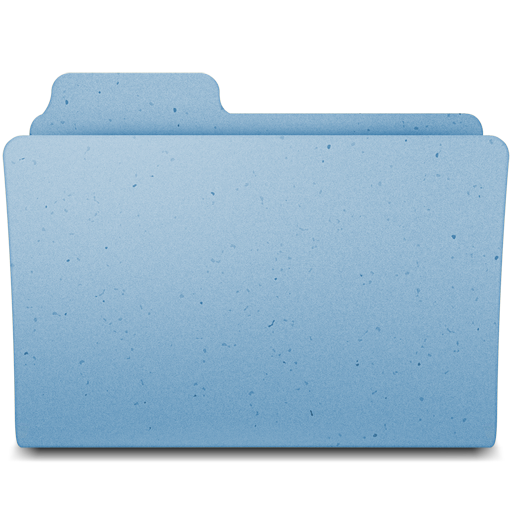
Full Code In Media Fire
http://bit.ly/NJqpAb
Subscribe to:
Posts (Atom)