If we develop applications ( XAML based) we have a problem with see the data in design view. Every application become successful when its interface is attractive. If our application is based on internet or any other computational task there is difficulty on make Interfaces without running the application real time. Here is solution for it
Use design time data binding which already with the xaml based application
Today we going to develop the windows 8.1 store application. ( This is same with any XAML based Application )
1. Create Windows 8.1 store application form the Visual studio. (I named it as DesignTimedata )
2. Create ViewModel to bind the run time data , In following here is My MainViewModel.cs
With this example we are not going to the use internet or other tasks .therefore just hard corded the values in constructor
2. Then bind the ViewModel to the View with relevant tags.
You can use Singleton or Page Resource binding . In here Im using Bind the ViewModel to the Page in XAML . It gives me intellisense in XAML .
Here is my MainPage.xaml
Here is my design time ViewModel. It will contains all test data which displayed in design view in visual studio
4. Then lets bind the design time data into the design (xaml) . In here there is always tag like this
With this d we can define all the properties which page have and change them. but none of them are effecting the real application .
Lets bind the ViewModel to the page and use it with design time . Here is my full page
Now we can see the vaues that we put to test the Application UI and make any changes to it.
Here is runtime result of the app
Enjoy
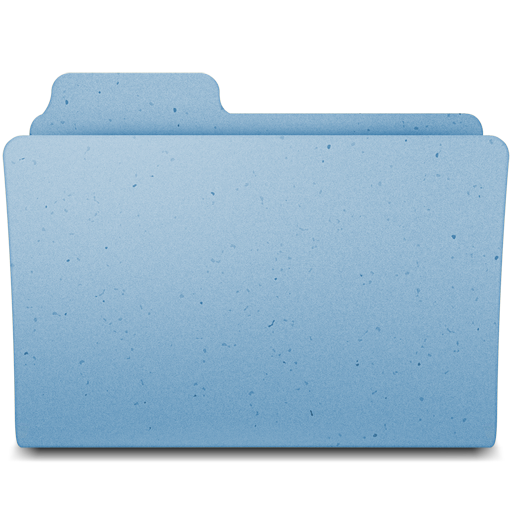
Full Code
http://bit.ly/1xiJ3yZ
Use design time data binding which already with the xaml based application
Today we going to develop the windows 8.1 store application. ( This is same with any XAML based Application )
1. Create Windows 8.1 store application form the Visual studio. (I named it as DesignTimedata )
2. Create ViewModel to bind the run time data , In following here is My MainViewModel.cs
With this example we are not going to the use internet or other tasks .therefore just hard corded the values in constructor
namespace DesignTimeData.Runtime { public class MainViewModel:INotifyPropertyChanged { private string _Title { get; set; } public string Title { get { return _Title; } set { _Title = value; OnPropertyChanged("Title"); } } private string _Description { get; set; } public string Description { get { return _Description; } set { _Description = value; OnPropertyChanged("Description"); } } public MainViewModel() {
// Hard coded runtime data
this._Title = "Title in Run time"; this._Description = "Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Maecenas porttitor congue massa. Fusce posuere, magna sed pulvinar ultricies, purus lectus malesuada libero, sit amet commodo magna eros quis urna."; } // Create the OnPropertyChanged method to raise the event // Use in MVVM public event PropertyChangedEventHandler PropertyChanged; protected void OnPropertyChanged(string name) { PropertyChangedEventHandler handler = PropertyChanged; if (handler != null) { handler(this, new PropertyChangedEventArgs(name)); } } } }
2. Then bind the ViewModel to the View with relevant tags.
You can use Singleton or Page Resource binding . In here Im using Bind the ViewModel to the Page in XAML . It gives me intellisense in XAML .
Here is my MainPage.xaml
<Page x:Class="DesignTimeData.MainPage" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:local="using:DesignTimeData" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:runtime="using:DesignTimeData.Runtime" mc:Ignorable="d"> <Page.DataContext> <runtime:MainViewModel/> </Page.DataContext>
3 .Then Create New Class which have exact name of your view model. Better to use different namespace / folder . In this case It is MainViewModel Here is my design time ViewModel. It will contains all test data which displayed in design view in visual studio
namespace DesignTimeData.DesignTimedata { public class MainViewModel { public string Title { get { return "DesignTime Title"; } } public string Description { get { return "Design time description"; } } } }
4. Then lets bind the design time data into the design (xaml) . In here there is always tag like this
mc:Ignorable="d"with every page. something defines under this tag will not be displayed in the runtime. then this is the one that we need to use.
With this d we can define all the properties which page have and change them. but none of them are effecting the real application .
Lets bind the ViewModel to the page and use it with design time . Here is my full page
<Page x:Class="DesignTimeData.MainPage" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:local="using:DesignTimeData" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:desgn="using:DesignTimeData.DesignTimedata" xmlns:runtime="using:DesignTimeData.Runtime" mc:Ignorable="d"> <Page.DataContext> <runtime:MainViewModel/> </Page.DataContext> <d:Page.DataContext> <desgn:MainViewModel /> </d:Page.DataContext> <Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}"> <StackPanel Margin="100,150,0,0"> <TextBlock Style="{StaticResource HeaderTextBlockStyle}" Text="{Binding Title}"/> <TextBlock Style="{StaticResource SubtitleTextBlockStyle}" Text="{Binding Description}"/> </StackPanel> </Grid> </Page>
Now we can see the vaues that we put to test the Application UI and make any changes to it.
Here is runtime result of the app
Enjoy
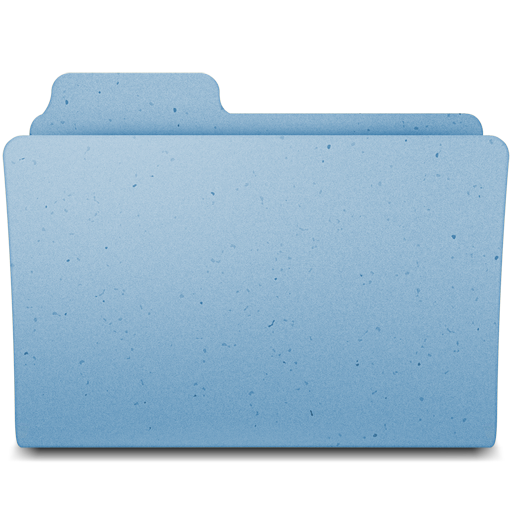
Full Code
http://bit.ly/1xiJ3yZ
0 comments:
Post a Comment