This post is related with the post that I put few months ago, If you don't have already configured Mongodb on the machine Here is the post that I put
Big question ?? How we use Mongo With C#? Is it hard?
Its really easy to use Mongo in .NET ,
Here im going to create Asp.NET Web API project for demonstration. (You can use any type of project )
Then Install official MongoDb Driver for .NET it name as MongoCsharpDriver Nuget package
Here is screenshot of my Mongo Database and document that I'm going to use
Here is the class I created for mongo operations
**This code is use latest standards of official mongo driver up to today
Then use this class with the code. That's It
Enjoy :)
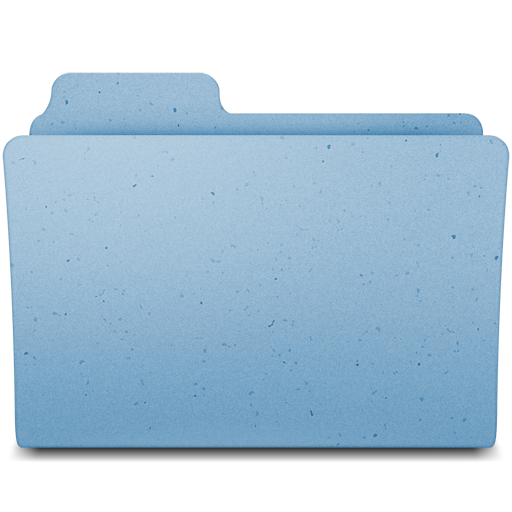
Full Code
http://bit.ly/MongoCSharp
Big question ?? How we use Mongo With C#? Is it hard?
Its really easy to use Mongo in .NET ,
Here im going to create Asp.NET Web API project for demonstration. (You can use any type of project )
Then Install official MongoDb Driver for .NET it name as MongoCsharpDriver Nuget package
Here is screenshot of my Mongo Database and document that I'm going to use
Here is the class I created for mongo operations
using MongoDB.Bson; using MongoDB.Driver; using System; using System.Collections.Generic; using System.Linq; using System.Web; namespace MongoDb.Models.MongoModels { public class MongoConnection { private MongoServer _ServerConnection { get; set; } public MongoDatabase _Database { get; set; } public string _ConnectionString { get { return "Server=localhost:27017"; } } public MongoConnection() { MongoClient client = new MongoClient(_ConnectionString); this._ServerConnection = client.GetServer(); ////////////////////Old way of create server//////////////////// //this._ServerConnection=MongoServer.Create(_ConnectionString); //Set the database this._Database = _ServerConnection.GetDatabase("DocumentOrDb"); } /// <summary> /// Get all data in the document /// </summary> /// <returns></returns> public MongoCursor<BsonDocument> GetAllUserdata() { return _Database.GetCollection<BsonDocument>("UserDetails").FindAll(); } /// <summary> /// Insert Item /// </summary> /// <param name="name"></param> /// <param name="address"></param> /// <returns></returns> public BsonDocument Insert(string name, string address) { MongoCollection<BsonDocument> UserDetails = _Database.GetCollection<BsonDocument>("UserDetails"); BsonDocument NewRecord = new BsonDocument() { { "Name",name}, {"Address",address} }; UserDetails.Insert(NewRecord); //return last inserted element return UserDetails.FindAll().Last(); } } }
**This code is use latest standards of official mongo driver up to today
Then use this class with the code. That's It
Enjoy :)
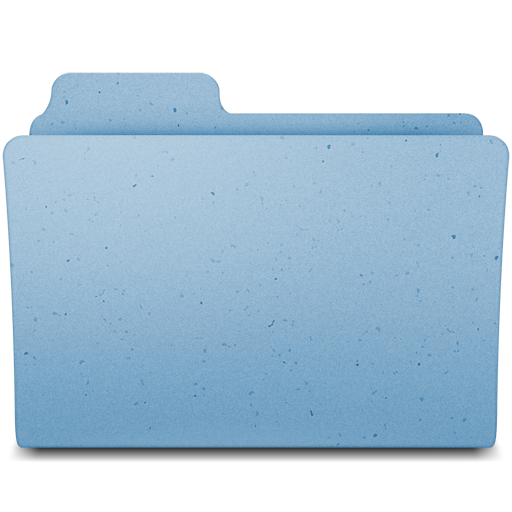
Full Code
http://bit.ly/MongoCSharp
0 comments:
Post a Comment