With my previous post about Creating Cloud Service I mentioned two Roles in Azure.
1. Web Role
2. Worker Role
With this Post we will working with Worker Role . I'm using the same code that I use in Creating Cloud Service post
What is Worker Role ??
Worker role is the component that doing background operations in Azure web service. If you need to implement background service such as background listener with your cloud service this is the component for you.
With physically Worker role is the Virtual machine which running the Server OS (Windows Server 2012 R2) without configuring IIS.( check the Web Role definition in Creating Cloud Service ).
Actually both of the Web Role and Worker Roles are derived from RoleEntryPoint Base class.
According to the OOP principles, yes Web Role can do the same Task that Worker Role can do. ( I hope to make discussion about it with later post ). We just give the chance to handle Web related tasks to web role and use worker role to handle non web based tasks. Because Web role may be over loaded when it try to handle all within it. Then Web role is free to handle more web based requests with more speed.
Lets Code ,
1. Open the code in visual studio and check it is working (Creating Cloud Service). Then go to the solution explorer
2. On the AzureServiceDemo -> Roles -> R - click -> New Worker Role Project
Then Select Worker Role and give name.. I'm giving PrabathslWorkerRole as name
After add the worker role you can see that it will add Worker Role project to your solution ans that project contains only one class called WorkerRole.cs
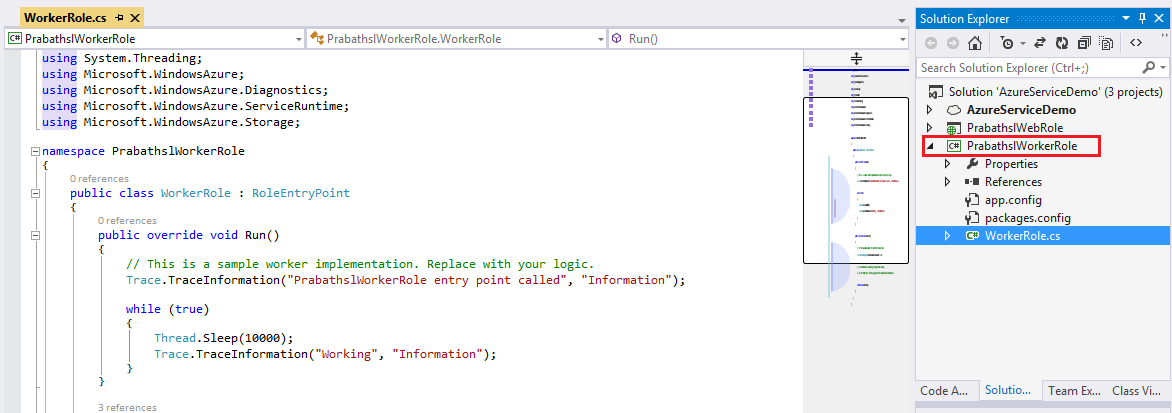
I'll add that class here for explanation
Simply public override bool OnStart() method is called when Role Started then it called public override void Run() Method. Run method should contain what we need to do in background. In here I'm going to configure HTTP listener.It take some inputs and generate output. If it needed to do repeatedly you need to add it inside forever while (true) loop
Also web role can forward jobs to the Worker role and Worker Role can also get inputs directly by it self. For that we can also insert our WCF service i worker role as well .
Replace the Run() with following code to listen port=4488 with HTTP protocol ...
This is local for the Azure VM.
Currently our VM's are haven't any external access.To allow access to the worker role we need change some configurations.
That called input end point. Lets define input endpoints. it allows traffic to go through our VM
3. Goto AzureServiceDemo Solution and Roles then double click on PrabathslWorkerRole
4. Click on Endpoints -> Add Endpoint button and add endpoint. I named it as prabathslEndpoint
External clients use this port to send traffic to service using public port
actually they send traffic through this port . Here is how people send traffic http://mysitedomain:4080/
Azure load balancer send the traffic (re route the traffic to Worker Role) through the private port to the Virtual Machine that run the worker role (4488)
**You can use any port as you wish
Now we are done and run it ..
Here is what i get when Listen to localhost
(http://127.0.0.1:4080/) We need to listen public port
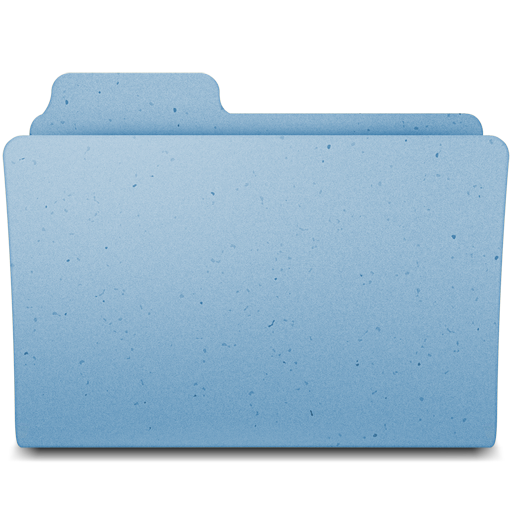
Full Code In Media Fire
http://bit.ly/worker_role
1. Web Role
2. Worker Role
With this Post we will working with Worker Role . I'm using the same code that I use in Creating Cloud Service post
What is Worker Role ??
Worker role is the component that doing background operations in Azure web service. If you need to implement background service such as background listener with your cloud service this is the component for you.
With physically Worker role is the Virtual machine which running the Server OS (Windows Server 2012 R2) without configuring IIS.( check the Web Role definition in Creating Cloud Service ).
Actually both of the Web Role and Worker Roles are derived from RoleEntryPoint Base class.
According to the OOP principles, yes Web Role can do the same Task that Worker Role can do. ( I hope to make discussion about it with later post ). We just give the chance to handle Web related tasks to web role and use worker role to handle non web based tasks. Because Web role may be over loaded when it try to handle all within it. Then Web role is free to handle more web based requests with more speed.
Lets Code ,
1. Open the code in visual studio and check it is working (Creating Cloud Service). Then go to the solution explorer
2. On the AzureServiceDemo -> Roles -> R - click -> New Worker Role Project
Then Select Worker Role and give name.. I'm giving PrabathslWorkerRole as name
After add the worker role you can see that it will add Worker Role project to your solution ans that project contains only one class called WorkerRole.cs
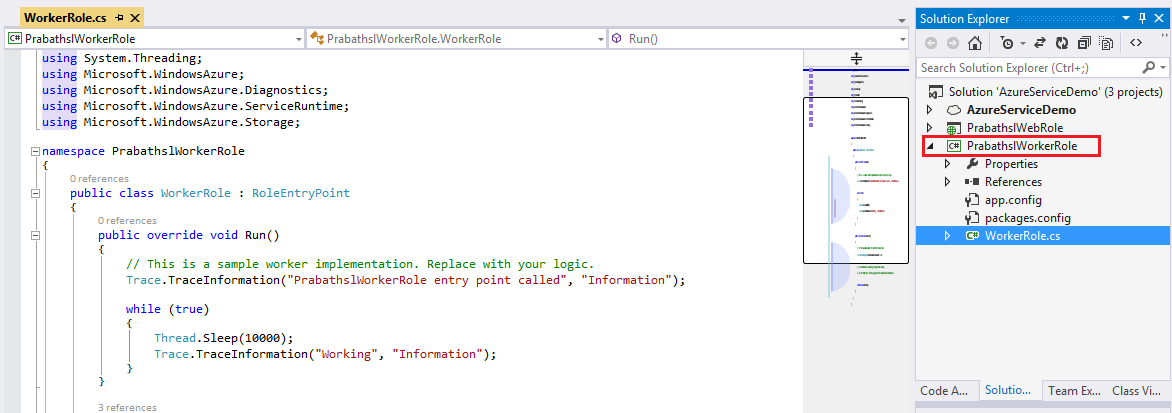
I'll add that class here for explanation
using
System;
using
System.Collections.Generic;
using
System.Diagnostics;
using
System.Linq;
using
System.Net;
using
System.Threading;
using
Microsoft.WindowsAzure;
using
Microsoft.WindowsAzure.Diagnostics;
using
Microsoft.WindowsAzure.ServiceRuntime;
using
Microsoft.WindowsAzure.Storage;
namespace
PrabathslWorkerRole
{
public
class
WorkerRole : RoleEntryPoint
{
public
override
void
Run()
{
// This is a sample worker implementation. Replace with your logic.
Trace.TraceInformation(
"PrabathslWorkerRole entry point called"
,
"Information"
);
while
(
true
)
{
Thread.Sleep(10000);
Trace.TraceInformation(
"Working"
,
"Information"
);
}
}
public
override
bool
OnStart()
{
// Set the maximum number of concurrent connections
ServicePointManager.DefaultConnectionLimit = 12;
// For information on handling configuration changes
// see the MSDN topic at http://go.microsoft.com/fwlink/?LinkId=166357.
return
base
.OnStart();
}
}
}
Simply public override bool OnStart() method is called when Role Started then it called public override void Run() Method. Run method should contain what we need to do in background. In here I'm going to configure HTTP listener.It take some inputs and generate output. If it needed to do repeatedly you need to add it inside forever while (true) loop
Also web role can forward jobs to the Worker role and Worker Role can also get inputs directly by it self. For that we can also insert our WCF service i worker role as well .
Replace the Run() with following code to listen port=4488 with HTTP protocol ...
public
override
void
Run()
{
HttpListener listner =
new
HttpListener();
//This will ping to Http://myserviceurl:4488 port
listner.Prefixes.Add(
"http://+:4488/"
);
listner.Start();
//Set the response to transformable format
string
responseMessage = "<html><title>Response from Worker</title><body>
<h1>Prabathsl.blogspot.com</h1></body></html>";
byte
[] buffer = Encoding.UTF8.GetBytes(responseMessage);
while
(
true
)
{
HttpListenerContext context = listner.GetContext();
//then set the response
HttpListenerResponse response = context.Response;
response.ContentLength64 = buffer.Length;
Stream output = response.OutputStream;
//write output stream
output.WriteAsync(buffer, 0, buffer.Length);
output.Close();
}
}
This is local for the Azure VM.
Currently our VM's are haven't any external access.To allow access to the worker role we need change some configurations.
That called input end point. Lets define input endpoints. it allows traffic to go through our VM
3. Goto AzureServiceDemo Solution and Roles then double click on PrabathslWorkerRole
4. Click on Endpoints -> Add Endpoint button and add endpoint. I named it as prabathslEndpoint
External clients use this port to send traffic to service using public port
actually they send traffic through this port . Here is how people send traffic http://mysitedomain:4080/
Azure load balancer send the traffic (re route the traffic to Worker Role) through the private port to the Virtual Machine that run the worker role (4488)
**You can use any port as you wish
Now we are done and run it ..
Here is what i get when Listen to localhost
(http://127.0.0.1:4080/) We need to listen public port
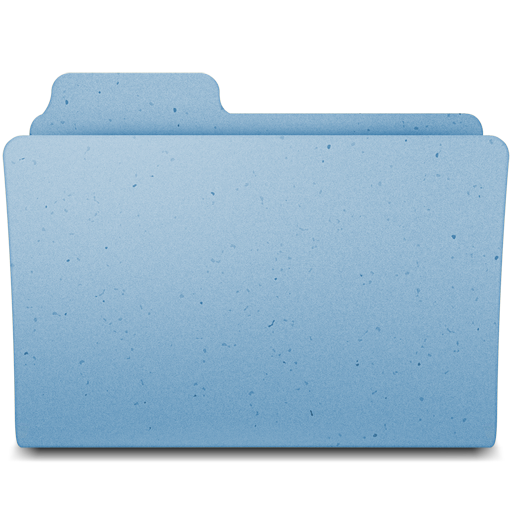
Full Code In Media Fire
http://bit.ly/worker_role
0 comments:
Post a Comment